C++ and Linear Algebra
category: general [glöplog]
When it comes Graphics Programming and making Demos, what are some of the important concept, or areas of focus for C++ and Linear Algebra that I should perhaps pay special attentions to in order to ensure a solid foundation for graphics programming in the future?
(especially C++, since it encompasses so much and there's essentially no end to its mastery.)
And, I'm probably getting ahead of myself here, but I figured I would ask just out of curiosity. What actually determines the size of demo? Just the amount of text/lines of codes a source files contains? or do other things play a factor as well? such as, limiting the amount of variables and functions, chaining statements into one whenever possible,etc ?
Are there any books or articles I should read up for writing clean, efficient code or on reducing the program's size?
-----------------------------------------------------------------------------------------------
Currently I'm learning C++ from:
Book: C++ Primer 5th Edition
website: http://www.learncpp.com/
(which is quite the perfect supplement to the book above and sometimes even provide more examples.)
For Linear Algebra:
starting with:
Introduction to Linear Algebra by Gilbert Strang
so far, everything is going well, and I'm really enjoying the whole learning process :)
On top of that, seeing new demos and interesting topics being discussed on this site only fuels the fire even more! ^_^
(especially C++, since it encompasses so much and there's essentially no end to its mastery.)
And, I'm probably getting ahead of myself here, but I figured I would ask just out of curiosity. What actually determines the size of demo? Just the amount of text/lines of codes a source files contains? or do other things play a factor as well? such as, limiting the amount of variables and functions, chaining statements into one whenever possible,etc ?
Are there any books or articles I should read up for writing clean, efficient code or on reducing the program's size?
-----------------------------------------------------------------------------------------------
Currently I'm learning C++ from:
Book: C++ Primer 5th Edition
website: http://www.learncpp.com/
(which is quite the perfect supplement to the book above and sometimes even provide more examples.)
For Linear Algebra:
starting with:
Introduction to Linear Algebra by Gilbert Strang
so far, everything is going well, and I'm really enjoying the whole learning process :)
On top of that, seeing new demos and interesting topics being discussed on this site only fuels the fire even more! ^_^
This may be slightly controversial; but I'd suggest that you watch Casey Muratoris handmade hero stream, and maybe his C introduction streams as well.
You didn't give much information about your background, but if you're not an experienced programmer already, that might give you some context on how to do the work of writing demos, and how to start, and keep going, and a good mindset for it. Even though Casey doesn't work specifically on demos, I think his process transfers well. I wish I had seen this 15 years ago, would have saved me a lot of trouble.
You didn't give much information about your background, but if you're not an experienced programmer already, that might give you some context on how to do the work of writing demos, and how to start, and keep going, and a good mindset for it. Even though Casey doesn't work specifically on demos, I think his process transfers well. I wish I had seen this 15 years ago, would have saved me a lot of trouble.
Linear algebra has nothing to do with C++. Just pick up a good maths book, or go to a university or something like that, or start from here. I learned mine from Erwin Kreyszig's Advanced Engineering Mathematics.
Quote:
And, I'm probably getting ahead of myself here, but I figured I would ask just out of curiosity. What actually determines the size of demo? Just the amount of text/lines of codes a source files contains? or do other things play a factor as well? such as, limiting the amount of variables and functions, chaining statements into one whenever possible,etc ?
It's a combination of: amount of code, number of external library calls you do, how much data and especially how diverse the data that you stuff into the binary is. Most importantly, however, you should have a good idea about how your compression algorithm works, so you can structure everything in a way that the compressor can deal with.
Ryg gave an excellent talk on that subject 10 years ago, and it is still 100% valid today: http://www.farbrausch.de/~fg/seminars/workcompression.html
+1 for handmade hero. He has prettty strong opinions on a lot of things though, which is good to keep in mind.
revival, I also recommend his streams nowadays to anyone starting in programming.. I wish I had it as source materials 10yo
As Preacher said: c++ has nothing to do with linear algebra.
If you need math in your code, simply include "math.h" and use its functions.
If you need math in your code for small prods <=64k, you should use intrinsics, which is almost as talking to the CPU itself as it is x86-code. Atleast in Visual Studio you can easily inline assembler-code.
Wikipedia about Intrinsics
And from my 4k-basecode what i am using:
Ignore those "#pragma"-lines, they are just for Crinkler so it knows what kind of data is in the next block of code. Keep them lines if you use crinkler, tho. ;)
RNG is no intrinsic-code btw, but maybe you´ll need it.
You need to do it this way to avoid including the whole math.dll (by including math.h) in your final executable, which is in the megabytes iirc, so absolutely impossible to get under 64k while using it.
If you need math in your code, simply include "math.h" and use its functions.
If you need math in your code for small prods <=64k, you should use intrinsics, which is almost as talking to the CPU itself as it is x86-code. Atleast in Visual Studio you can easily inline assembler-code.
Wikipedia about Intrinsics
And from my 4k-basecode what i am using:
Code:
//___sInUs___
#pragma code_seg(".crtemuf")
float ASMsinf(float i)
{ __asm fld i
__asm fsin
}
//___cOsInUs___
#pragma code_seg(".crtemuf")
float ASMcosf(float i)
{ __asm fld i
__asm fcos
}
//___rOUnd_flOAt_tO_IntEgEr___
#pragma code_seg(".crtemuf")
int ASMlrintf (float flt)
{ int reti;
__asm
{
fld flt
fistp reti // rounds ;)
}
return reti;
}
//___sqUArE_rOOt___
#pragma code_seg(".crtemuf")
float ASMsqrtf(float i)
{ __asm fld i
__asm fsqrt
}
//___mOdUlO_flOAt___
#pragma code_seg(".crtemuf")
float ASMfmodf(float i, float j)
{ __asm fld j
__asm fld i
__asm fprem
__asm fxch
__asm fstp i
}
//___AbsOlUtE_flOAt___
#pragma code_seg(".crtemuf")
float ASMfabsf(float i)
{ __asm fld i
__asm fabs
}
//___clEAr_mEmOry___
#pragma code_seg(".crtemui")
void ASMZeroMemory(void* dest, SIZE_T s)
{ __asm mov edi, dest
__asm xor eax, eax
__asm mov ecx, s
__asm rep stosb
}
//___cOpy_mEmOry___
#pragma code_seg(".crtemui")
void ASMCopyMemory(void* dest, void* souAe, SIZE_T s)
{ __asm mov esi, souAe
__asm mov edi, dest
__asm mov ecx, s
__asm rep movsb
}
//___rAndOm_nUmbEr_gEnErAtOr___
#pragma data_seg(".rand")
DWORD RandSeed;
#pragma code_seg(".crtemui")
unsigned long random()
{
RandSeed = (RandSeed * 196314165) + 907633515;
return RandSeed;
}
Ignore those "#pragma"-lines, they are just for Crinkler so it knows what kind of data is in the next block of code. Keep them lines if you use crinkler, tho. ;)
RNG is no intrinsic-code btw, but maybe you´ll need it.
You need to do it this way to avoid including the whole math.dll (by including math.h) in your final executable, which is in the megabytes iirc, so absolutely impossible to get under 64k while using it.
so sorry for the confusion Preacher, Hardy.
I should've phrased the title better.
I was just asking what key concept or topics in Linear Algebra and C++ are most important when it comes to using them and applying them for graphics programming. Especially, C++ since it encompasses such wide array of topics and is used for so many things.
sorry hardy! :)
I should've phrased the title better.
I was just asking what key concept or topics in Linear Algebra and C++ are most important when it comes to using them and applying them for graphics programming. Especially, C++ since it encompasses such wide array of topics and is used for so many things.
sorry hardy! :)
You need to know matrices from linear algebra. They are often used in computer graphics, e.g. for transformations (rotating, zooming etc.).
As much as I remember, linear algebra was a subject of the first semester of studying computer science at university where you took the exam and which you never needed again, except maybe to understand some concepts of theoretical computer science better.
As much as I remember, linear algebra was a subject of the first semester of studying computer science at university where you took the exam and which you never needed again, except maybe to understand some concepts of theoretical computer science better.
Small Demos Are about being zipped and coded with reused functions to be zippable.
They also use Compiler settings optimized for small binaries with null redundancy.
Small Demos Are about using methods that on runtime create an array of floats to Point at wich as few bits as possible.
Using small functions that recursicely define long not too repetitive strings to be parsed as instructions for other Things. Its similar to seeding conways game of life with 10 dots to run for 300 cycles just to read a section from that for any purpose.
Small Demos utilize self similarity of fractals and procedural textures like simplex noise.
Small Demos use libraries that now every graphic card has. Some use functions to assemble audio from Images or to seed Images from audio. Pointers Are small. Libraries that tjey Point to Are much larger.
Small Demos use More coarse interpolations of splines for movement.
Small Demos prefer cubic objects because abstraction is key anyways and fighting the uncanny valley in <8 k is barely worth it.
Small Demos Are about compressing object detail. Dont even bother with tesselation when a signed distance function results in infinitely smooth curves with very Little code.
Small Demos Are about not using Software libraries but instead copying from libraries The barr necessities.
They also use Compiler settings optimized for small binaries with null redundancy.
Small Demos Are about using methods that on runtime create an array of floats to Point at wich as few bits as possible.
Using small functions that recursicely define long not too repetitive strings to be parsed as instructions for other Things. Its similar to seeding conways game of life with 10 dots to run for 300 cycles just to read a section from that for any purpose.
Small Demos utilize self similarity of fractals and procedural textures like simplex noise.
Small Demos use libraries that now every graphic card has. Some use functions to assemble audio from Images or to seed Images from audio. Pointers Are small. Libraries that tjey Point to Are much larger.
Small Demos use More coarse interpolations of splines for movement.
Small Demos prefer cubic objects because abstraction is key anyways and fighting the uncanny valley in <8 k is barely worth it.
Small Demos Are about compressing object detail. Dont even bother with tesselation when a signed distance function results in infinitely smooth curves with very Little code.
Small Demos Are about not using Software libraries but instead copying from libraries The barr necessities.
Adok: Linear algebra is super-useful for multivariate statistics, including machine learning.
ollj:
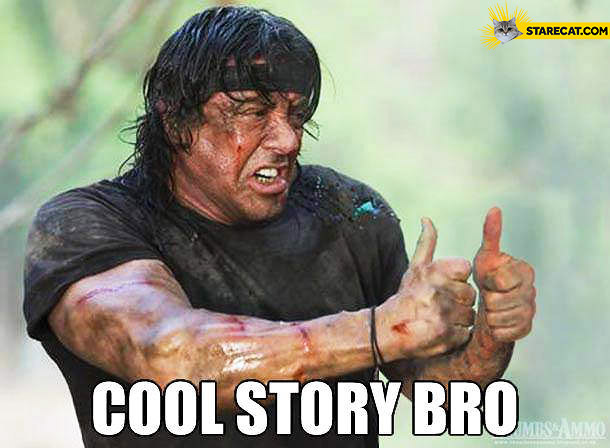
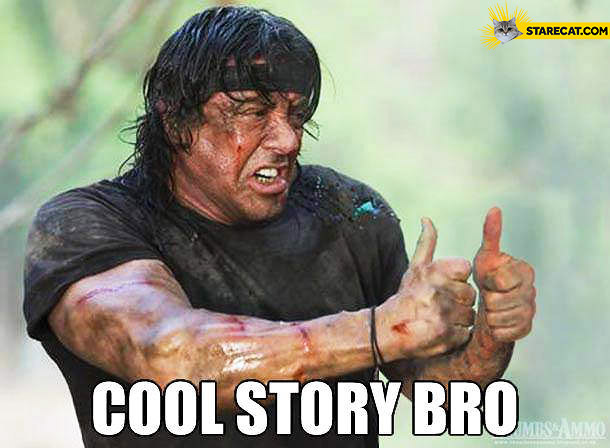
As far as 3D graphics and linear algebra is concerned, you'll need polynoms, spaces, vectors, matrices, trigonometry and all the geometric interpretations of it (then again, of all people I interviewed for a graphics programmer position, only _one_ was able to answer "what's the difference between a dot product and a cross product?". Humans. *sigh*). Add imaginary/complex numbers and quaternions to the mix, and you have the tool set to solve the vast majority of graphics problems. Being fluent in exponential functions and logarithms can't hurt either. Differentiation and integration are somewhat important too but you can mostly navigate around them by solving stuff numerically and/or making them magically disappear thanks to discrete steps. :)
Also don't shy away from looking behind the curtain. As a practical example the smoothstep function which eases in and out a gradient between 0 and 1 can be easily conjured out of thin air just by 10th grade math: "I need something that goes from 0 to 1 and eases in and out. So, f(0)=0, f(1)=1 and the derivatives at the end should be flat, so f'(0)=f'(1)=0. These are four constraints so a cubic polynomial should do". The rest is a sheet of paper and 5 minutes of Gaussian elimination :)
Then there's the vast undocumented and entirely unscientific part that I like to call "demoscene math" which involves creative mixing of whatever math function is available to make something look good. But that comes with experience. Just lerp, smooth, pow, abs and sin your way out of everything :D
Also don't shy away from looking behind the curtain. As a practical example the smoothstep function which eases in and out a gradient between 0 and 1 can be easily conjured out of thin air just by 10th grade math: "I need something that goes from 0 to 1 and eases in and out. So, f(0)=0, f(1)=1 and the derivatives at the end should be flat, so f'(0)=f'(1)=0. These are four constraints so a cubic polynomial should do". The rest is a sheet of paper and 5 minutes of Gaussian elimination :)
Then there's the vast undocumented and entirely unscientific part that I like to call "demoscene math" which involves creative mixing of whatever math function is available to make something look good. But that comes with experience. Just lerp, smooth, pow, abs and sin your way out of everything :D
If there was a subject “demoscene math”, Navis would be the professor.
Personally, I don't think you need to learn matrices. You just need to know how to build them and how they're used. Knowing how they work doesn't hurt, though, but most of the time you'll be using them as black boxes anyway.
Another tip: be sure to fully understand the relationship of sin, cos, tan and the unit circle formula. Based on this itˋs super easy to understand 2d rotation matrices/transforms. This is the basis of all 3d rotation math and many more topics (e.g. FOV calculations, mesh deformation effects, ...)
Also, "sin your way out of everything" is pretty good life advice in general ;)
Quote:
handmade hero
After watching an hour or two of the very beginning (the C ones), I think this is a) an example of why video tutorials are not the end-all-be-all approach to teaching and b) more interesting as a business model (patreon+segue to game pre-orders) than as a teaching tool.
Quote:
Just lerp, smooth, pow, abs and sin your way out of everything :D
Add atan2 and you're golden.

Quote:
Another tip: be sure to fully understand the relationship of sin, cos, tan and the unit circle formula.
Also, understand the relationship of dot/cross products and sin/cos. Additionally what normals are usefu for. And if you know how all that relates to the various entries in a matrix you´ve already covered >90% of your daily 3d code needs :)
Tomaes, don't discount it so quickly. There is value in just seeing him work his way out (and justifying his solutions) of problems. I.e. in the tactical act of working on code, something that's really hard to capture in writing and very rarely ever done. (The kind of things you learn by osmosis when working with colleagues)
The episode guide might be better for catching up:
https://hero.handmadedev.org/jace/guide/
The episode guide might be better for catching up:
https://hero.handmadedev.org/jace/guide/
Also it's certainly not done to earn money, the game isn't even his main game, which is this one http://mollyrocket.com/news_0023.html in a complete other genre.
Add Bezier curves to your mathematical arsenal. Just because they are sexy!
About the size of demos, the most important factor is data, not code. To make small demos, we minimize the data and try to generate most of it procedurally. And then an executable file cruncher, especially tailored to code compression, do the job.
This is a grosso modo explanation. The devil, and the fun, is in the details. The more devils, the less kilobytes. ;]
About the size of demos, the most important factor is data, not code. To make small demos, we minimize the data and try to generate most of it procedurally. And then an executable file cruncher, especially tailored to code compression, do the job.
This is a grosso modo explanation. The devil, and the fun, is in the details. The more devils, the less kilobytes. ;]
BTW, there was a beginner's course on Linear Algebra in Hugi #23, written by iliks:
http://www.hugi.scene.org/online/coding/hugi%2023%20-%20illa1.htm
http://www.hugi.scene.org/online/coding/hugi%2023%20-%20illa1.htm
thank you sir ! bookmarked :)